Hasura
This tutorial assumes Hasura backend was selected. If Apollo Server Express was selected, check out the Apollo Server Express version.
In this tutorial we'll add an optional due date for todos.
Learn how to:
- Navigate your full stack
- Make cross platform changes
Backend
Spin up Hasura and Postgres locally. From the root of the project run:
This runs docker-compose up
which brings up everything in Docker (Hasura, Postgres). Check out the docker-compose.yaml file and the docs for details.
Bring up the Hasura developer console. This is where we can make changes to Hasura and Postgres.
Add a "date" column to todos.
- Click "DATA" on the top navigation bar
- Click "todos" under tables on the left
- Click the "Modify" tab under "todos"
- Click "Add a new column"
- Set "column name" to "date"
- Set "column_type" to "Date"
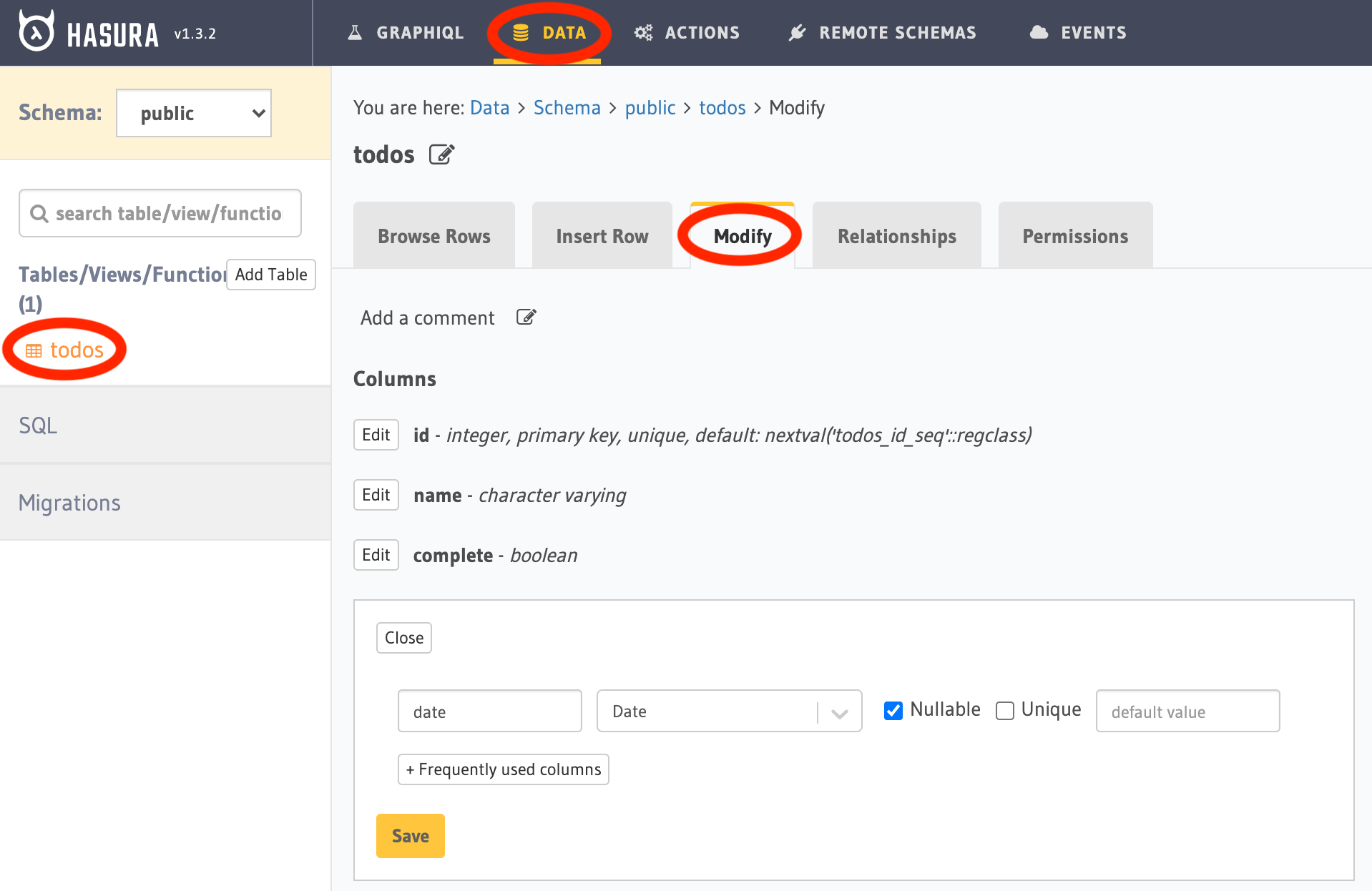
Learn more on the Hasura schema docs
Add insert permissions for "date".
- Click the "Permissions" tab under "todos"
- Click the filter icon under the "anonymous" role ("user" if auth is enabled) and the "insert" permissions
- Click "Column insert permissions"
- Check "date"
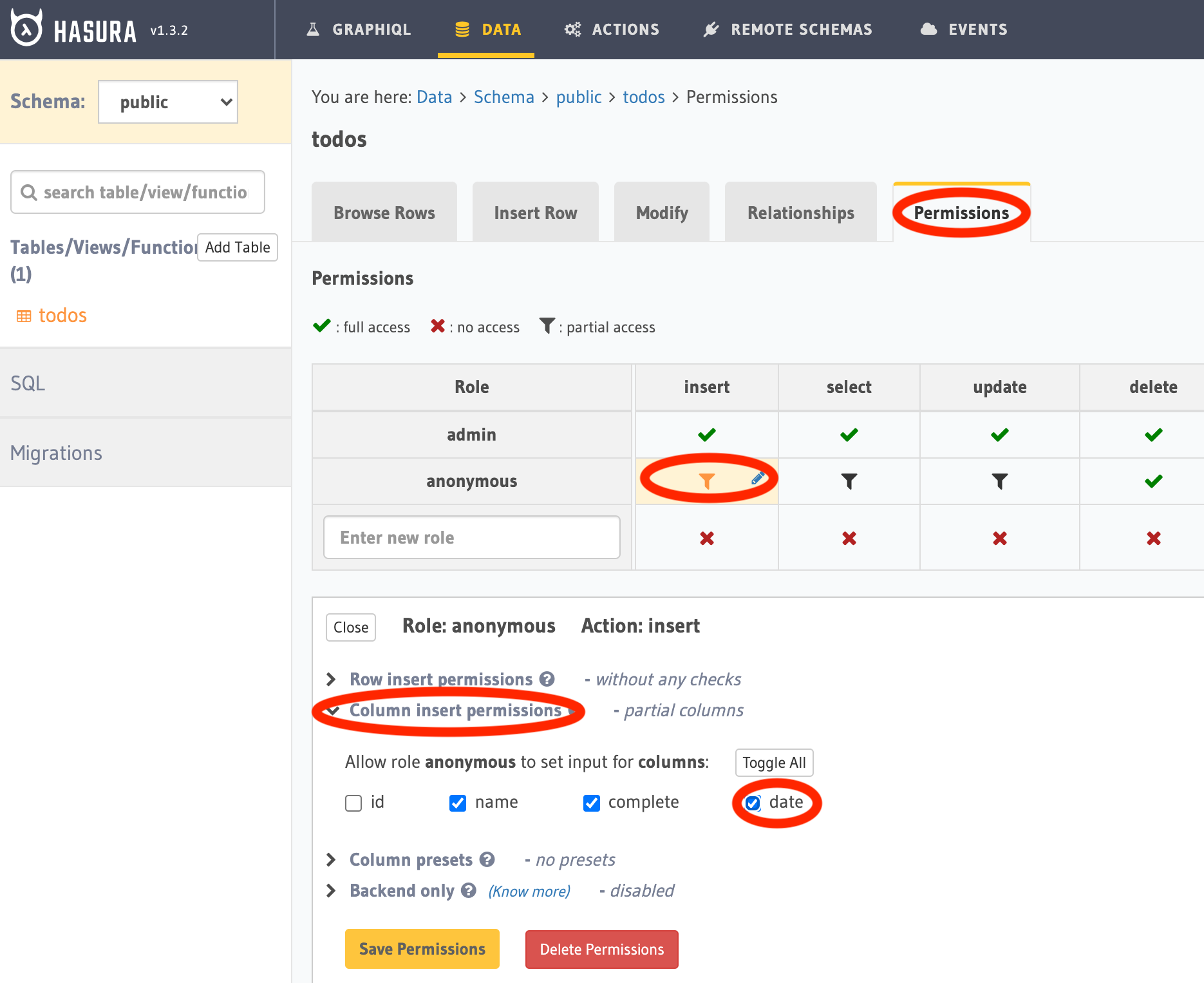
Add select permissions for "date".
- Click the filter icon under the "anonymous" role ("user" if auth is enabled) and the "select" permissions
- Click "Column select permissions"
- Check "date"
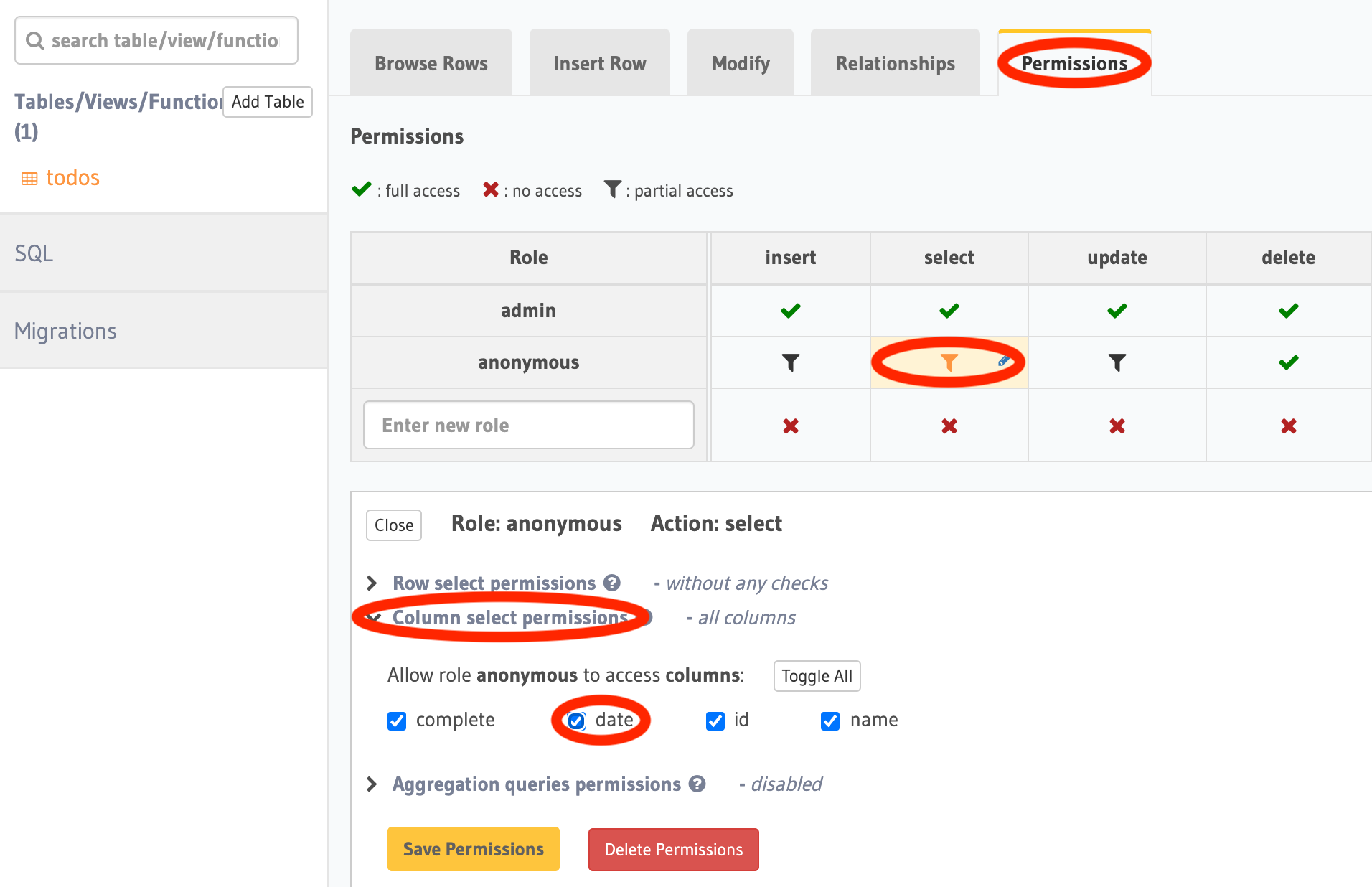
That's it!
If you run git status
you'll notice that changes have been made to the hasura/metadata/
and hasura/migrations/
folders. This tracks changes to Hasura and should be checked in. Learn more about CFS Hasura migrations on the docs.
Common
Common contains shared code across the full stack. It's used for client Apollo GraphQL requests from both web and mobile.
Update the GraphQL query and create mutation requests to include "date".
In packages/common/src/graphql/todos.graphql
make the following changes:
That's it!
When your run yarn start
from the root of the project TS code is generated and packages/common
is built automatically. See graphql-code-generator to learn how this works.
Web
If web was included, then follow these steps to configure it for the new todo date field.
We're using the Material-UI Pickers library for the date picker. This has a couple dependencies.
First, install the packages:
Add MuiPickersUtilsProvider
to packages/web/src/App.tsx
:
This tells pickers which date management library it should use with MuiPickersUtilsProvider. This component takes a utils prop, and makes it available down the React tree with React Context. It should be used at the root of your component tree, or at the highest level you wish the pickers to be available.
Update packages/web/src/components/CreateTodo.tsx
:
Update ListItemText
in packages/web/src/components/Todo.tsx
:
Make sure files are properly formatted and linted. In VSCode, with the recommended extensions, this happens automatically. Otherwise, from the root of the project run:
With the full stack running (yarn start
from root), navigate to http://localhost:3000. You should see your new todo date field! 🎉
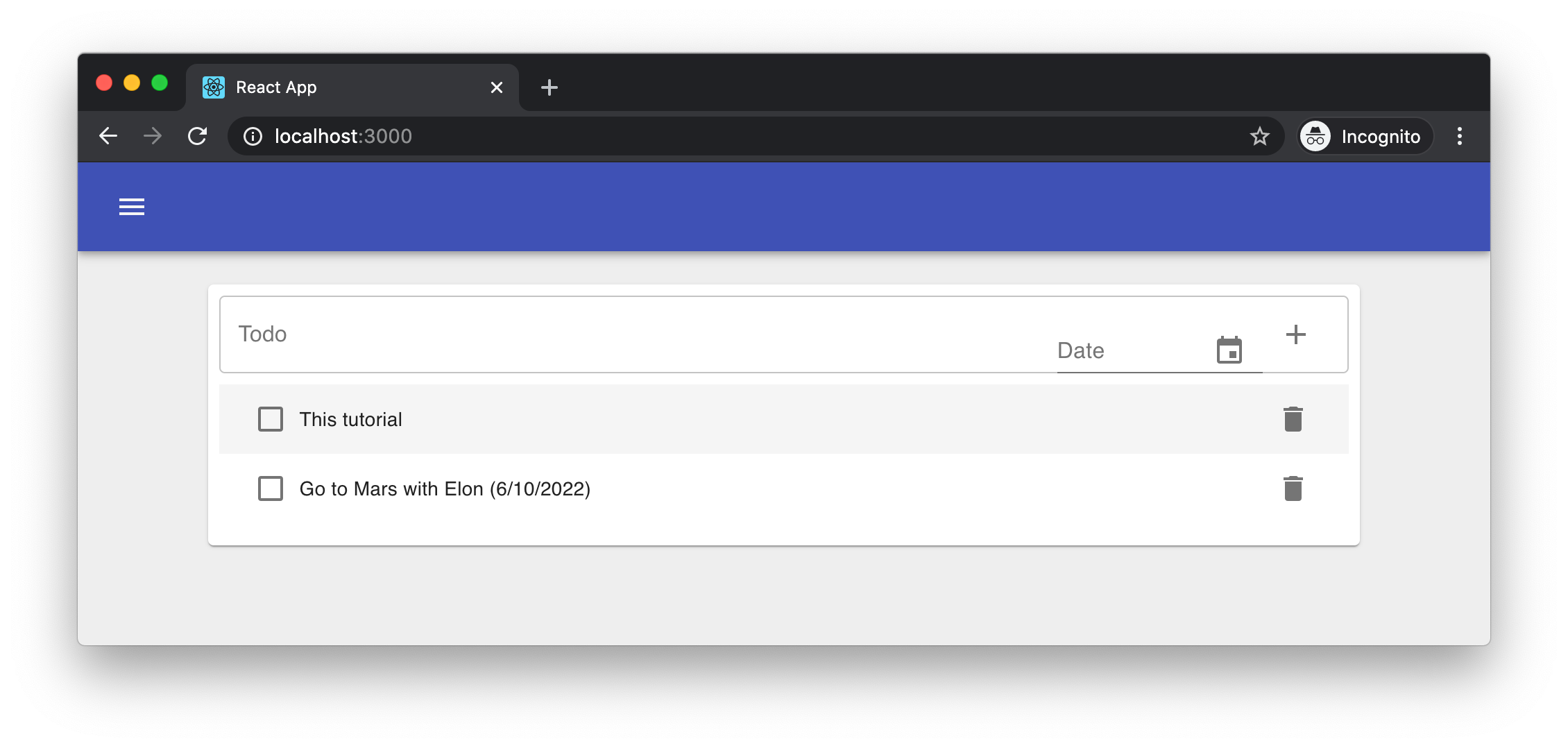
Mobile
If mobile was included, then follow these steps to configure it for the new todo date field.
We're using the react-native-modal-datetime-picker for the date picker.
First, install the package and dependency:
We're using yarn expo install
to ensure Expo supported versions of the libraries are used. Since Expo uses native code in its SDK, typically only a single version of a library with native dependencies can be used per version of Expo. Expo handles this mapping with its install command. Learn more on the Expo docs.
Update packages/mobile/src/components/CreateTodo.tsx
:
Update ListItem.Title
in packages/mobile/src/components/Todo.tsx
:
Make sure files are properly formatted and linted. In VSCode, with the recommended extensions, this happens automatically. Otherwise, from the root of the project run:
With the full stack running (yarn start
from root), navigate to http://localhost:19002 and bring up the application. You should see your new todo date field! 🎉
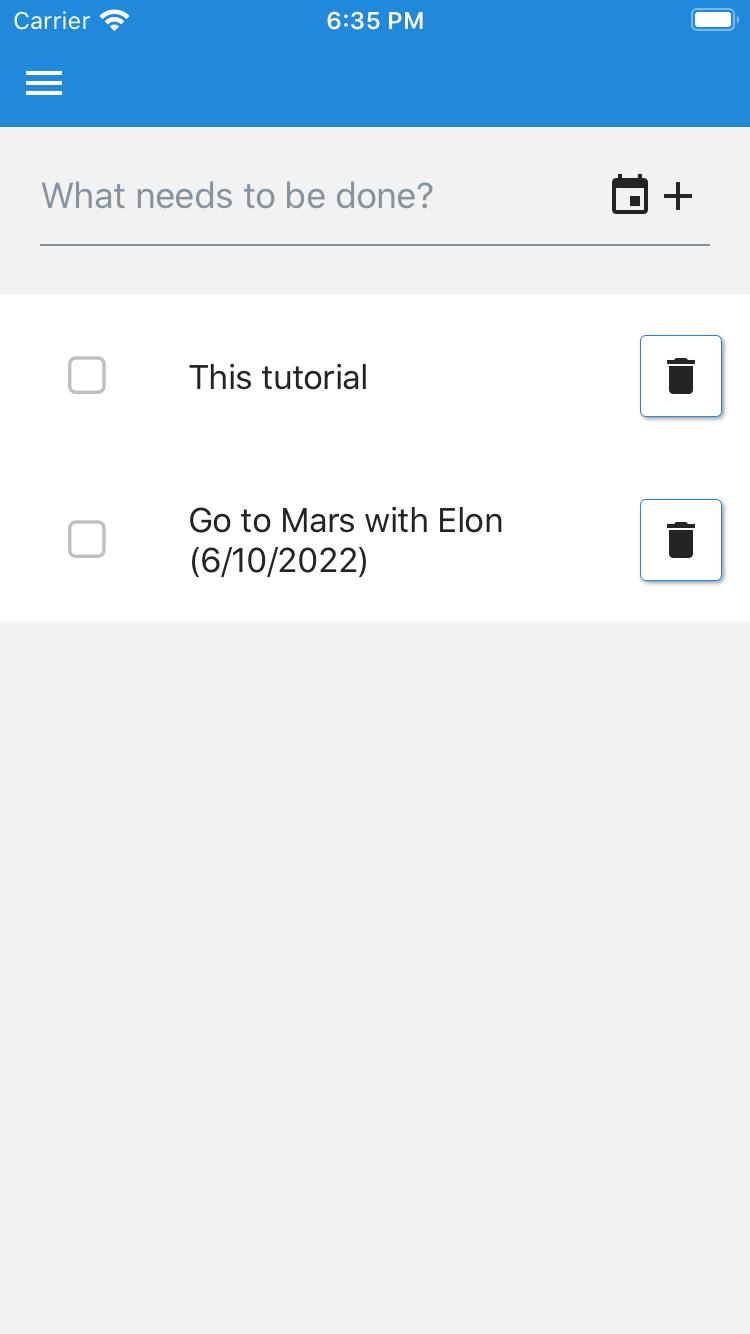