Apollo Server Express
This tutorial assumes Apollo Server Express backend was selected. If Hasura was selected, check out the Hasura version.
In this tutorial we'll add an optional due date for todos.
Learn how to:
- Navigate your full stack
- Make cross platform changes
Backend
We need to add the date column to Postgres and include it in the schema. The GraphQL resolver is written in a generic enough way that it will handle this change without modification.
Add the date column to packages/server/src/entity/Todo.ts
:
packages/server/src/entity/Todo.ts
contains the Postgres definition for the todos
table. This is called a TypeORM "entity". Entity is a class that maps to a database table.
Update the GraphQL schema at packages/server/src/graphql/schema.ts
:
packages/server/src/graphql/schema.ts
contains the GraphQL schema that Apollo Server Express uses. Your GraphQL server uses a schema to describe the shape of your data graph. This schema defines a hierarchy of types with fields that are populated from your back-end data stores. The schema also specifies exactly which queries and mutations are available for clients to execute against your data graph.
That's it!
Since synchronize: true
is set in packages/server/ormconfig.js
, changes to packages/server/src/entity/Todo.ts
will automatically sync to the database. Learn more about migrations on the TypeORM docs. To see how GraphQL requests are resolved check out packages/server/src/getResolvers.ts
and the resolver docs.
Common
Common contains shared code across the full stack. It's used for client Apollo GraphQL requests from both web and mobile. It's also used on the Apollo Server Express backend for testing.
Update the GraphQL query and create mutation requests to include "date".
In packages/common/src/graphql/todos.graphql
update to:
That's it!
When your run yarn start
from the root of the project TS code is generated and packages/common
is built automatically. See graphql-code-generator to learn how this works.
Web
If web was included, then follow these steps to configure it for the new todo date field.
We're using the Material-UI Pickers library for the date picker. This has a couple dependencies.
First, install the packages:
Add MuiPickersUtilsProvider
to packages/web/src/App.tsx
:
This tells pickers which date management library it should use with MuiPickersUtilsProvider. This component takes a utils prop, and makes it available down the React tree with React Context. It should be used at the root of your component tree, or at the highest level you wish the pickers to be available.
Update packages/web/src/components/CreateTodo.tsx
:
Update ListItemText
in packages/web/src/components/Todo.tsx
:
Make sure files are properly formatted and linted. In VSCode, with the recommended extensions, this happens automatically. Otherwise, from the root of the project run:
With the full stack running (yarn start
from root), navigate to http://localhost:3000. You should see your new todo date field! ๐
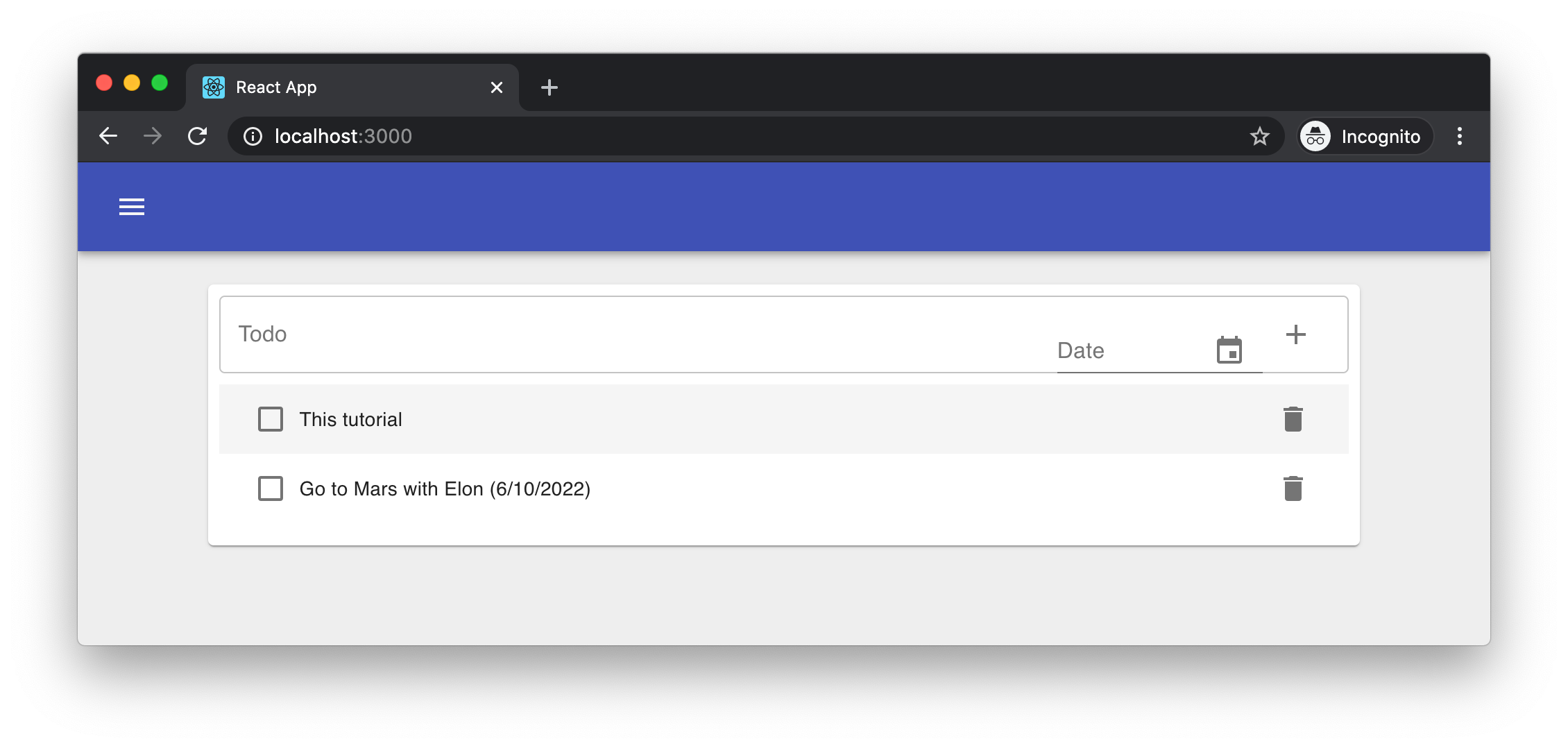
Mobile
If mobile was included, then follow these steps to configure it for the new todo date field.
We're using the react-native-modal-datetime-picker for the date picker.
First, install the package and dependency:
We're using yarn expo install
to ensure Expo supported versions of the libraries are used. Since Expo uses native code in its SDK, typically only a single version of a library with native dependencies can be used per version of Expo. Expo handles this mapping with its install command. Learn more on the Expo docs.
Update packages/mobile/src/components/CreateTodo.tsx
:
Update ListItem.Title
in packages/mobile/src/components/Todo.tsx
:
Make sure files are properly formatted and linted. In VSCode, with the recommended extensions, this happens automatically. Otherwise, from the root of the project run:
With the full stack running (yarn start
from root), navigate to http://localhost:19002 and bring up the application. You should see your new todo date field! ๐
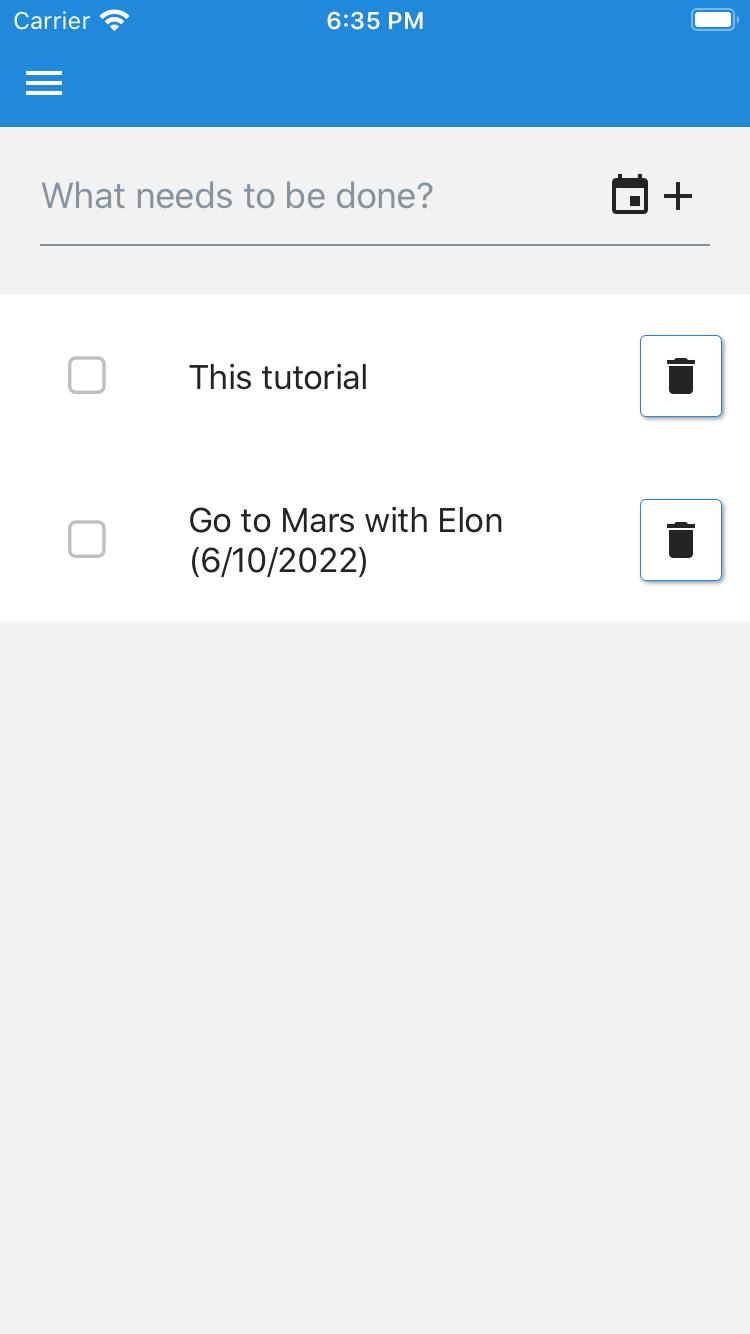